What is a JavaScript Prototype?
Prototype is a property of an object in JavaScript, which is important when considering the concept of inheritance. Inheritance, in JavaScript, results in properties or functions from an ancestor (or prototype) being passed on to objects created from its blueprint. We can manipulate the behaviors of an object by defining properties on the prototype of the object's constructor. Here is an example of how inheritance works, using the prototype property.
function SnarkyMessage(lovelyMessage) {
this.lovelyMessage = lovelyMessage;
}
var javaScriptRules = new SnarkyMessage("I love JavaScript");
javascriptRules.lovelyMessage
SnarkyMessage.prototype.snark = function () {
this.lovelyMessage += " sucks");
};
javascriptRules.snark();
javascriptRules.lovelyMessage
Let's break this down into parts and talk about what's going on in detail. First, I'm creating a constructor function called SnarkyMessage, which takes a string and assigns it to a property, lovelyMessage.
function SnarkyMessage(lovelyMessage) {
this.lovelyMessage = lovelyMessage;
}
Then I define a variable, javaScriptRules, using the constructor from above. Calling the constructor property of javaScriptRules in Chrome DevTools can help illustrate this.
var javaScriptRules = new SnarkyMessage("I love JavaScript");
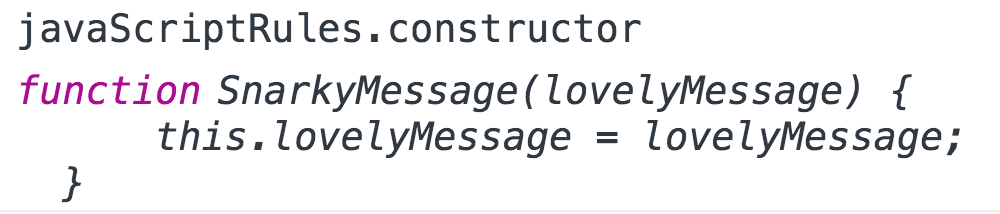
As you can see, javaScriptRules.lovely message is set to the string passed to constructor, "I love JavaScript"
javascriptRules.lovelyMessage // this outputs "I love JavaScript"
Now I'm going to add a function, snark, to the prototype of javaScriptRules by defining it via the constructor's prototype property. The function snark will append the word "sucks" to the lovelyMessage property of SnarkyMessage objects.
SnarkyMessage.prototype.snark = function () {
this.lovelyMessage += " sucks");
};
When we call snark on javaScriptRules, we will "snarkify" javaScriptRules as a result of using the prototype property on its constructor, SnarkyMessage. Thus javaScriptRules.lovelyMessage has now inherited the properties of snark, and it throws a snarky addition on the end of lovelyMessage.
javascriptRules.snark();
javascriptRules.lovelyMessage // will output "I love JavaScript sucks"
Note that if we tried to define snark on SnarkyMessage, and not on the SnarkyMessage prototype, running the program raises the following error message: TypeError: javaScriptRules.snark is not a function. This indicates that using the prototype property is necessary for javaScriptRules to inherit the snark function. In sum, the big takeaway from this exercise is that in JavaScript, prototype is used to apply inherited properties to an object. JavaScript objects are still pretty new to me, and this post made me think long and hard about objects and inheritance. I'm excited to keep learning more!